What are the implications of leveraging computational tools for targeted coding practices? Understanding the role of these specialized coding methods is crucial for modern software development.
The term refers to individuals specializing in software development who employ sophisticated, often automated, methods to efficiently produce code. These methods can include automated code generation tools, libraries for specific tasks, and specific programming paradigms (e.g., functional programming) for optimizing code. For instance, a programmer using a library to generate database interaction code rapidly is utilizing principles associated with this specialized approach. Another example would be using a code generator to create UI elements.
Such specialized approaches significantly boost developer productivity by automating routine tasks, allowing developers to focus on higher-level design and problem-solving. This, in turn, leads to faster project completion times, reduced development costs, and ultimately, increased application quality by reducing potential errors in code generated automatically, though thorough testing remains critical. The historical context involves the increasing complexity of software, demanding quicker development cycles and greater code quality. This specialized approach is a direct response to that demand.
Moving forward, exploring the specific techniques and tools employed by these individuals, the methodologies they follow, the programming languages utilized, and the resultant improvements in code quality are vital for understanding the broader implications of this development in software engineering.
Code Optimization Strategies
Efficient coding practices are crucial for modern software development. Understanding the techniques employed by specialized coders is essential for maximizing productivity and quality.
- Automated code generation
- Optimized algorithms
- Modular design
- Testing frameworks
- Version control
- Performance analysis
- Debugging methodologies
These aspects collectively contribute to enhanced code quality and reduced development time. Automated code generation, for example, speeds up repetitive tasks, allowing developers to focus on more complex aspects. Modular design enhances maintainability, while performance analysis ensures optimized execution. Effective testing frameworks prevent bugs early in the development cycle. A robust understanding and application of these methodologies is vital for producing reliable and scalable software applications. Version control systems, like Git, facilitate collaborative work and streamline code management. This integrated approach underlies modern software development practices.
1. Automated Code Generation
Automated code generation is a core component of the practices employed by individuals specializing in efficient code production. This automation streamlines tasks, significantly impacting development workflows. The process involves tools and systems that produce code from a specified set of inputs, ranging from user interface design specifications to database schemas. This automation frees developers from repetitive coding tasks, enabling focus on higher-level design elements and complex problem-solving. Examples include generating boilerplate code for database interactions, UI components, or specific algorithms. This automation, when applied correctly, can substantially reduce development time and potential errors.
The practical significance of automated code generation lies in its ability to improve development efficiency. By automating mundane tasks, developers can allocate more time to design, testing, and debugging, ultimately leading to better software quality. This translates to faster project completion times and reduced development costs. Furthermore, automated code often adheres to established coding standards, fostering consistency within large projects. Consider the impact of automatically generating code for a vast web applicationthe volume and complexity of manual coding would be prohibitive. Automating the creation of essential code elementsrouting, security layers, and communication protocols, for exampleis vital to project viability.
In conclusion, automated code generation is a critical tool in optimizing modern software development. Its use directly benefits the efficiency and quality of code, ultimately streamlining the entire process. However, developers must still possess a deep understanding of the underlying principles and ensure proper verification of generated code, highlighting the importance of a skilled human component alongside the automation.
2. Optimized Algorithms
Optimized algorithms are fundamental to the work of individuals specializing in efficient code production. Their proficiency involves selecting and refining algorithms to minimize resource consumption (time and memory) and maximize the speed and performance of code. This focus directly impacts the efficiency of software development and the quality of the resulting applications. The connection lies in the direct application of these algorithms by specialized coders to improve the performance and efficiency of the codebase.
- Algorithm Selection and Refinement
Expertise in algorithm selection is crucial. Coders must understand the strengths and weaknesses of various algorithms and choose the most appropriate one for a given task. This often involves evaluating the time and space complexities of algorithms. Refinement goes beyond selectionit often involves tailoring existing algorithms or designing new ones that leverage specific characteristics of the platform or data to optimize performance. Examples include using a more efficient sorting algorithm for large datasets or designing a custom search algorithm for specialized data structures.
- Data Structures and Algorithms
Understanding and applying appropriate data structures is intrinsically linked to algorithm optimization. A poorly chosen data structure can severely hinder the performance of an algorithm, while a suitable one can enhance speed and efficiency dramatically. Coders need to know when to utilize hash tables for fast lookups, trees for hierarchical data, or linked lists for dynamic insertions and deletions. This understanding is integral to generating efficient and well-performing code.
- Space Complexity Considerations
Efficient code prioritizes minimizing memory usage, known as space complexity. Coders must carefully consider data storage mechanisms and algorithm design choices to ensure efficient memory management. This impacts the scalability of applications, particularly in handling large volumes of data. Examples include choosing data structures that minimize redundant data storage or designing algorithms to avoid unnecessary temporary variable allocations.
- Time Complexity Analysis
Optimizing algorithms often involves evaluating the time complexity of different approaches and choosing the most time-efficient solution. This process often entails analyzing the steps within an algorithm and estimating the number of operations performed as the input size increases. The selection of a more optimized algorithm can result in dramatically reduced execution times, which is critical for real-time applications and responsive systems.
In summary, optimized algorithms form a cornerstone of effective software development. Specialized coders, or "icode fishers," demonstrate expertise in algorithm selection, refinement, and implementation, leading to faster, more efficient, and more robust applications. Their proficiency in understanding and utilizing appropriate data structures and analyzing time/space complexity underscores the importance of optimized algorithms in producing high-quality software.
3. Modular Design
Modular design, a cornerstone of software architecture, directly impacts the efficiency and effectiveness of specialized coding practices. Its application by "icode fishers" enhances code organization, maintainability, and reusability. This structured approach allows for the development of robust and scalable software systems.
- Improved Maintainability and Modification
Modular design facilitates easier maintenance and modification of software. Individual modules, like self-contained units, can be updated or replaced without affecting other parts of the system. This reduces the risk of introducing errors during maintenance and allows for quicker responses to changing requirements. In complex systems, the ability to isolate and address issues within specific modules dramatically increases the efficiency of troubleshooting and code refinement.
- Enhanced Reusability and Scalability
Modules often contain reusable components, which can be incorporated into different parts of the system or even other projects. This fosters a culture of code reuse, minimizing redundancy and maximizing efficiency. By promoting modularity, specialized coders can create components that readily scale with expanding functionalities or data volumes. This approach proves invaluable for adapting to the evolving needs of software systems. The reuse of validated code modules ensures stability and lowers the chance of introducing errors.
- Reduced Development Time and Cost
By compartmentalizing functions into independent modules, tasks become more manageable. This division of labor and the potential for parallel development can significantly shorten development timelines. The reusability of modules allows for faster integration into new projects and systems, further reducing the overall development cost. Smaller, more manageable components foster a more organized development environment, allowing teams to concentrate on specific modules simultaneously. Specialized coders contribute significantly by efficiently managing and leveraging these modular components.
- Enhanced Collaboration and Communication
Modular design promotes collaboration among developers working on different modules. Clearer definitions of responsibilities and interaction points streamline communication and prevent conflicts. This increased clarity facilitates a structured approach to development, fostering better team coordination and reducing the likelihood of errors arising from a lack of communication in large projects. Specialized coding emphasizes these collaborative advantages, creating stronger, more robust software.
In essence, modular design, as employed by "icode fishers," streamlines the development process by improving code organization, reusability, and maintainability. This, in turn, results in higher-quality software, faster development cycles, and enhanced collaboration among developers. The ability to effectively apply these principles is a core aspect of the specialized skills associated with efficient coding practices.
4. Testing Frameworks
Testing frameworks are integral to the work of individuals specializing in efficient code production. Robust testing is crucial for ensuring software quality and reliability, a direct concern of those focused on streamlined and high-quality coding. The use of testing frameworks is not simply an add-on but a fundamental aspect of the development process for "icode fishers," reflecting a dedication to creating dependable software.
- Automated Testing Strategies
Testing frameworks facilitate automated testing, enabling the execution of numerous test cases swiftly and reliably. Automated test suites execute predefined tests repeatedly, helping to detect bugs early in development. This approach, crucial for "icode fishers," allows for continuous verification, minimizing costly and time-consuming rework later in the project. Examples include unit tests for individual components, integration tests verifying interactions between modules, and end-to-end tests simulating the entire application flow. These automate processes, improving overall code quality by identifying and fixing problems proactively.
- Standardized Testing Procedures
Testing frameworks provide standardized procedures for writing and executing tests, promoting consistency and repeatability. This is essential for maintaining a high standard of code quality. A common testing framework establishes a clear, understood structure, benefiting both developers and maintainers. This structured approach ensures that tests are not only effective but also easily understood and maintained throughout the lifespan of the software. The predictability of testing frameworks allows for more accurate estimations of development timelines, while fostering better collaborative development.
- Early Defect Detection
Early identification of defects is paramount in software development. Testing frameworks allow for early defect detection, minimizing the impact of fixing bugs in later stages of development. This structured and consistent testing is a hallmark of the approach taken by "icode fishers." Identifying issues early frequently saves significant time and resources compared to finding them closer to deployment. Comprehensive testing plans, facilitated by frameworks, lead to more reliable software, lowering the potential for application failures in production environments.
- Code Coverage Analysis
Frameworks often support code coverage analysis, enabling developers to ascertain the extent of their test suites' impact on the application's codebase. This metric quantifies how much of the code is exercised by the tests. Identifying gaps in coverage is crucial for "icode fishers," as it helps pinpoint areas needing more tests. This approach enhances code quality by revealing un-tested parts of the code, making the entire project more robust. High code coverage is a key indicator of a well-tested and reliable application.
In conclusion, testing frameworks are indispensable tools for specialized coders focused on producing high-quality code. The ability to automate testing, standardize procedures, ensure early defect detection, and analyze code coverage underscores the importance of these frameworks in the overall software development process, aligning directly with the goals of "icode fishers" and creating more reliable and maintainable software.
5. Version Control
Version control systems are essential for individuals focused on efficient and high-quality code production. These systems, exemplified by Git, are crucial components in the toolkit of those specializing in code optimization. Version control facilitates collaboration, tracks changes over time, and ultimately contributes to the reliability and maintainability of software. The ability to revert to previous versions, manage concurrent edits, and track authorship is fundamental to the discipline of producing high-quality code. A crucial aspect is the ability to preserve the integrity and history of a project, providing mechanisms for tracking and resolving conflicts arising from simultaneous edits.
Practical applications underscore the importance of version control. Consider a large software development project. Multiple developers working concurrently on different modules benefit immensely from a system capable of tracking changes. Version control systems allow for the resolution of conflicts between developers' contributions, preventing data loss or corruption, which might result from unsynchronized code changes. Moreover, the ability to revert to previous code versions is invaluable in case of errors or unforeseen complications. Detailed change logs provide a historical record of updates, facilitating easier debugging and maintenance, critical in projects with long lifecycles. By providing a clear audit trail of changes, version control enhances transparency and accountability in the development process. Real-world examples demonstrate the application's value in reducing risks associated with software development, ensuring the integrity of code, and minimizing delays related to code resolution.
In summary, version control systems empower efficient code production by facilitating collaboration, tracking changes, and ensuring code reliability. The ability to revert to earlier versions, manage concurrent edits, and track authorship is fundamental to maintaining high code quality. This discipline aligns with the fundamental principles of effective software engineering, highlighting its necessity in large and complex projects. The meticulous tracking of code changes through version control is a defining characteristic of professional development practices, fostering a culture of accountability, transparency, and collaboration. For "icode fishers," mastering version control translates directly to more efficient and robust code production.
6. Performance analysis
Performance analysis is a crucial component in the toolkit of those specializing in efficient code production. This process involves evaluating the speed, resource utilization, and overall efficiency of software applications. For individuals focused on optimization (often referred to as "icode fishers"), performance analysis is not an afterthought but an integral part of the development lifecycle. By rigorously analyzing performance characteristics, developers can identify bottlenecks, inefficiencies, and areas for improvement. Effective performance analysis is essential for producing applications that are not only functional but also scalable and responsive. Consider a web application handling millions of users: performance analysis is paramount in ensuring smooth operation and preventing slowdowns.
The practical application of performance analysis is diverse. For instance, in a database application, performance analysis might reveal slow query execution times. Identifying the specific queries causing bottlenecks allows developers to rewrite queries more efficiently, optimize database indexes, or modify the database schema for enhanced performance. Similarly, in a real-time application, analysis might uncover delays in data processing or communication bottlenecks. Addressing these issues often involves tuning algorithms, improving communication protocols, or optimizing caching strategies. In mobile app development, performance analysis could expose excessive memory consumption, leading developers to refactor code, minimize unnecessary object allocations, or optimize data structures for lower memory footprints. Real-world examples demonstrate that performance analysis, when applied systematically, directly translates to improved user experience, reduced operational costs, and enhanced software reliability.
In summary, performance analysis is a critical tool for individuals specializing in efficient code production. Its systematic application allows developers to identify and address performance bottlenecks, leading to optimized software applications. The proactive approach to performance tuning enhances not only the speed and responsiveness of the application but also its overall stability and long-term maintainability. This methodical approach is a core component of efficient coding practices, underpinning the creation of high-performance, scalable software systems and showcasing the vital role performance analysis plays in the work of "icode fishers."
7. Debugging Methodologies
Debugging methodologies are integral to the practice of efficient code production, a hallmark of "icode fishers." Effective debugging is not simply about identifying errors; it's a structured process emphasizing prevention and systematic resolution. A well-defined debugging approach can significantly reduce development time and enhance the reliability of software applications. This methodical approach is essential for isolating and fixing errors, preventing regressions, and ensuring quality in a rapidly evolving codebase.
The practice of debugging methodologies necessitates a combination of technical skills and a structured approach. Methodologies like the divide-and-conquer approach, in which the problem is broken down into smaller, more manageable parts, are valuable. Utilizing logging mechanisms to track program execution, or employing debuggers for step-by-step code inspection, enables identification of the root causes of issues. Systematic examination of error messages, meticulous analysis of code paths, and comprehensive testing strategies are all facets of a robust debugging process. Real-world examples abound. Consider a large-scale software project where multiple developers contribute code. A well-defined debugging methodology ensures efficient resolution of errors affecting shared components. In such scenarios, the methodical approach employed in debugging minimizes disruption to ongoing work and prevents errors from spreading. Similarly, a streamlined debugging methodology fosters the creation of maintainable and robust softwareessential in large-scale projects.
In essence, robust debugging methodologies are vital for "icode fishers." These structured approaches, including careful code review, thorough testing, and the use of debugging tools, are paramount in minimizing errors and improving the quality and efficiency of code production. The ability to debug effectively is not merely a technical skill but a crucial aspect of a "coder"s toolkit. The methodologies, from logging to breakpoints, promote a disciplined approach, contributing to the overall productivity and reliability of software systems. While challenges in debugging complex systems remain, the continued refinement and adoption of effective methodologies are fundamental to the development of high-quality software, crucial to the goals of those specializing in efficient code production.
Frequently Asked Questions about Specialized Coding Practices
This section addresses common inquiries regarding specialized coding practices, often referred to as "icode fishers" methodologies. These questions delve into the core concepts, tools, and benefits associated with these approaches.
Question 1: What are the primary tools and techniques used by "icode fishers"?
Specialized coders leverage a range of tools and techniques, including automated code generation tools, optimized algorithms, modular design principles, comprehensive testing frameworks, robust version control systems, and performance analysis methodologies. These approaches contribute to improved efficiency and enhanced code quality.
Question 2: How do specialized coding practices impact development speed and cost?
By automating repetitive tasks and optimizing code efficiency, specialized approaches generally shorten development cycles. Reduced errors through comprehensive testing and automated processes minimize the need for extensive rework, ultimately leading to lower overall project costs.
Question 3: What role does modular design play in these specialized approaches?
Modular design is crucial for maintainability and scalability in software. Breaking down complex systems into smaller, independent modules enhances code organization, promotes reuse, and streamlines the process of modifying or updating specific components without impacting the overall system.
Question 4: Why is automated testing so important in specialized coding?
Automated testing is essential for ensuring high-quality code. It proactively identifies defects and errors early in the development cycle, which leads to lower costs associated with fixes in later stages. This approach also minimizes regressions and enhances overall code reliability.
Question 5: How do these practices support scalability in software applications?
By employing optimized algorithms, modular design, and thorough testing, these techniques enable the development of software capable of handling increasing data volumes and user loads without compromising performance. This is particularly important in modern applications with substantial growth potential.
In summary, specialized coding practices, often exemplified by "icode fishers," prioritize code quality, efficiency, and long-term maintainability through strategic utilization of various tools and methodologies. These approaches address the challenges of developing complex and large-scale software systems.
Next, we'll delve deeper into the practical implementation of these methodologies within specific software development environments.
Conclusion
The exploration of specialized coding practices, often associated with the term "icode fishers," reveals a critical shift in modern software development. This approach emphasizes efficiency, quality, and maintainability, particularly within the context of complex systems. Key elements include streamlined automated processes for code generation, optimized algorithms for superior performance, modular design principles for enhanced maintainability, rigorous testing frameworks for error prevention, robust version control for collaboration and project integrity, and methodical performance analysis to ensure optimal application scaling. These practices contribute to faster development cycles, reduced costs, and higher-quality software. The ability to address large-scale development challenges through systematic application of these techniques underlines their importance in contemporary software engineering.
The future of software development likely hinges upon the continued refinement and adoption of these specialized coding methodologies. As software systems become increasingly complex and demanding, the ability to leverage optimized, robust, and maintainable solutions will be critical. Further research and development in these specialized areas will continue to drive innovation and efficiency in the field. A deeper understanding of these principles is essential for developers seeking to remain competitive and build reliable, scalable software for the future.

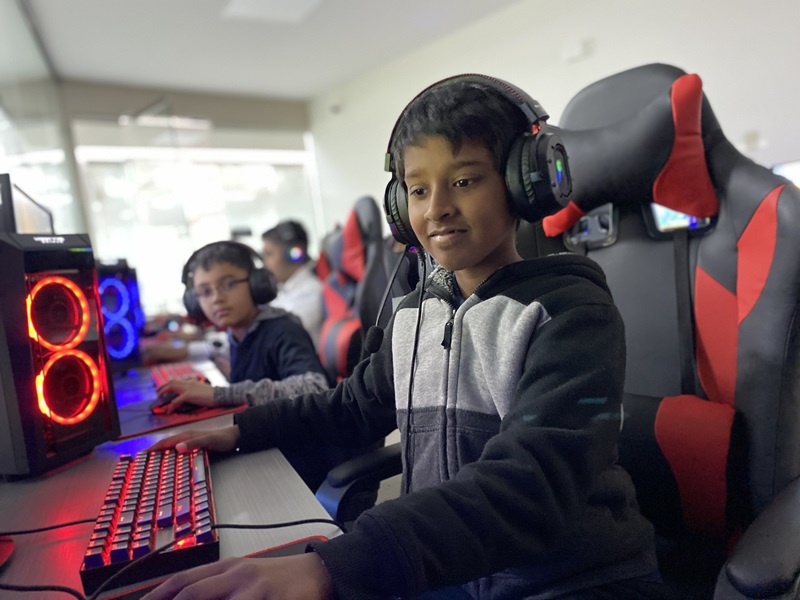
